C語言的那些小秘密之鏈表(二)
雖然兩個(gè)指針變量存放在不同的單元,但是它們都指向同一個(gè)存儲(chǔ)單元,所以在釋放的時(shí)候只能釋放一次,如果釋放兩次就會(huì)出錯(cuò)。注意切不可同時(shí)使用free(pointer_1);和 free(pointer_2);,否則將會(huì)出現(xiàn)內(nèi)存錯(cuò)誤。對(duì)出錯(cuò)原因不懂的可以參考我前面的文章《C語言的那些小秘密之指針》。這個(gè)代碼和我們雙鏈表的最大區(qū)別是它只能使用free()函數(shù)進(jìn)行一次釋放,而我們雙鏈表中看似使用了兩次,但實(shí)則一次而已。原因就在于我們?cè)趯?duì)頭結(jié)點(diǎn)分配空間的時(shí)候分配的是存放data指針變量的存儲(chǔ)空間(注意:所有的指針變量在分配的時(shí)候均分配4字節(jié)大小的存儲(chǔ)空間,如果有什么疑惑可以參考我之前寫的《C語言的那些小秘密之指針》)。所以釋放的時(shí)候也是釋放的存放指針變量data的存儲(chǔ)空間,并沒有釋放掉指針變量data所指向的存儲(chǔ)空間。所以在釋放data指向的存儲(chǔ)空間時(shí),我們只需要在main()函數(shù)中對(duì)stu[i]所指向的存儲(chǔ)空間使用free()函數(shù)即可,因?yàn)閐ata和它指向的是同一個(gè)存儲(chǔ)空間。
本文引用地址:http://m.butianyuan.cn/article/272382.htm接下來我們來添加一個(gè)在頭結(jié)點(diǎn)添加結(jié)點(diǎn)的模塊。
#include
#include
typedef enum _DListReturn
{
DLIST_RETURN_OK,
DLIST_RETURN_FAIL
}DListReturn;
typedef struct _DStu
{
int score;
}DStu;
typedef struct _DListNode
{
struct _DListNode* prev;
struct _DListNode* next;
DStu* data;
}DListNode;
typedef struct _DList
{
DListNode* head;
}DList;
typedef DListReturn (*DListPrintFunction)(void* data);
DListNode* dlist_node_create(void* data)
{
DListNode* node;
if((node = (DListNode*) malloc(sizeof(DListNode)))==NULL)
{
printf("分配空間失敗!");
exit(0);
}
if(node != NULL)
{
node->prev = NULL;
node->next = NULL;
node->data =(DStu*)data;
}
return node;
}
DList* dlist_head_create(void)
{
DList* thiz;
if((thiz = (DList*)malloc(sizeof(DList)))==NULL)
{
printf("分配空間失敗!");
exit(0);
}
if(thiz != NULL)
{
thiz->head = NULL;
}
return thiz;
}
DListReturn dlist_append(DList* thiz, void* data)
{
DListNode* node = NULL;
DListNode* cursor = NULL;
if((node = dlist_node_create(data)) == NULL)
{
return DLIST_RETURN_FAIL;
}
if(thiz->head == NULL)
{
thiz->head = node;
return DLIST_RETURN_OK;
}
cursor = thiz->head;
while(cursor != NULL && cursor->next != NULL)
{
cursor = cursor->next;
}
cursor->next = node;
node->prev = cursor;
return DLIST_RETURN_OK;
}
DListReturn dlist_prepend(DList* thiz, void* data)
{
DListNode* node = NULL;
DListNode* cursor = NULL;
if((node = dlist_node_create(data)) == NULL)
{
return DLIST_RETURN_FAIL;
}
if(thiz->head == NULL)
{
thiz->head = node;
return DLIST_RETURN_OK;
}
cursor = thiz->head;
if(thiz->head == cursor)
thiz->head = node;
node->next = cursor;
cursor->prev = node;
return DLIST_RETURN_OK;
}
DListReturn dlist_print(DList* thiz, DListPrintFunction print)
{
DListNode* iter = thiz->head;
while(iter != NULL)
{
print(iter->data);
iter = iter->next;
}
printf("n");
return DLIST_RETURN_OK;
}
DListReturn print_int(void* data)
{
DStu* ss=(DStu*)data;
printf("%dt ", ss->score);
return DLIST_RETURN_OK;
}
DListReturn dlist_node_destroy(DListNode* node)
{
if(node != NULL)
{
node->next = NULL;
node->prev = NULL;
free(node);
}
return DLIST_RETURN_OK;
}
DListReturn dlist_destroy(DList* thiz)
{
DListNode* iter = thiz->head;
DListNode* next = NULL;
while(iter != NULL)
{
next = iter->next;
dlist_node_destroy(iter);
iter = next;
}
thiz->head = NULL;
free(thiz);
return DLIST_RETURN_OK;
}
int main(int argc, char* argv[])
{
int i = 0;
DList* dlist = dlist_head_create();
for(i = 0; i < 7; i++)
{
DStu* stu =(DStu*) malloc(sizeof(DStu));
stu->score = i;
dlist_append(dlist, (void*)stu);
}
for(i = 0; i < 7; i++)
{
DStu* stu =(DStu*) malloc(sizeof(DStu));
stu->score = i;
dlist_prepend(dlist, (void*)stu);
}
dlist_print(dlist, print_int);
dlist_destroy(dlist);
return 0;
}
我們?cè)谏弦欢未a添加了紅色部分代碼后,使得其在已經(jīng)生成的雙鏈表的頭結(jié)點(diǎn)處添加結(jié)點(diǎn),運(yùn)行結(jié)果如下:
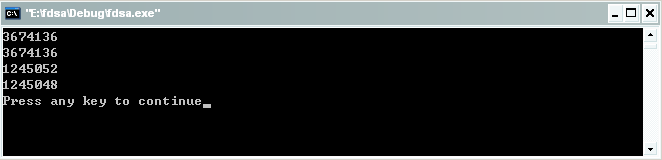
6 5 4 3 2 1 0 0 1 2
3 4 5 6
Press any key to continue
可以看出結(jié)果與我們的要求完全符合。如果你認(rèn)真分析了代碼的話你就會(huì)發(fā)現(xiàn),我們的兩種添加方式中有公共代碼出現(xiàn),那就說明我們的代碼可以繼續(xù)改進(jìn),把公共代碼放到一個(gè)函數(shù)中,讀者可以另外編寫一個(gè)函數(shù)來實(shí)現(xiàn),使得我們編寫的代碼得到充分的利用。
篇幅似乎有些過長(zhǎng)了,接下來我就不再一一講解了,我在這里只是想起一個(gè)引路的作用,讀者完全可以在此基礎(chǔ)之上繼續(xù)編寫雙鏈表其余部分的功能,其他的功能模塊讀者可以在此基礎(chǔ)上一一添加上去,到下一篇的時(shí)候我們將走進(jìn)linux內(nèi)核鏈表,繼續(xù)鏈表之旅的最后一站。由于本人水平有限,博客中的不妥或錯(cuò)誤之處在所難免,殷切希望讀者批評(píng)指正。同時(shí)也歡迎讀者共同探討相關(guān)的內(nèi)容,如果樂意交流的話請(qǐng)留下你寶貴的意見。
評(píng)論